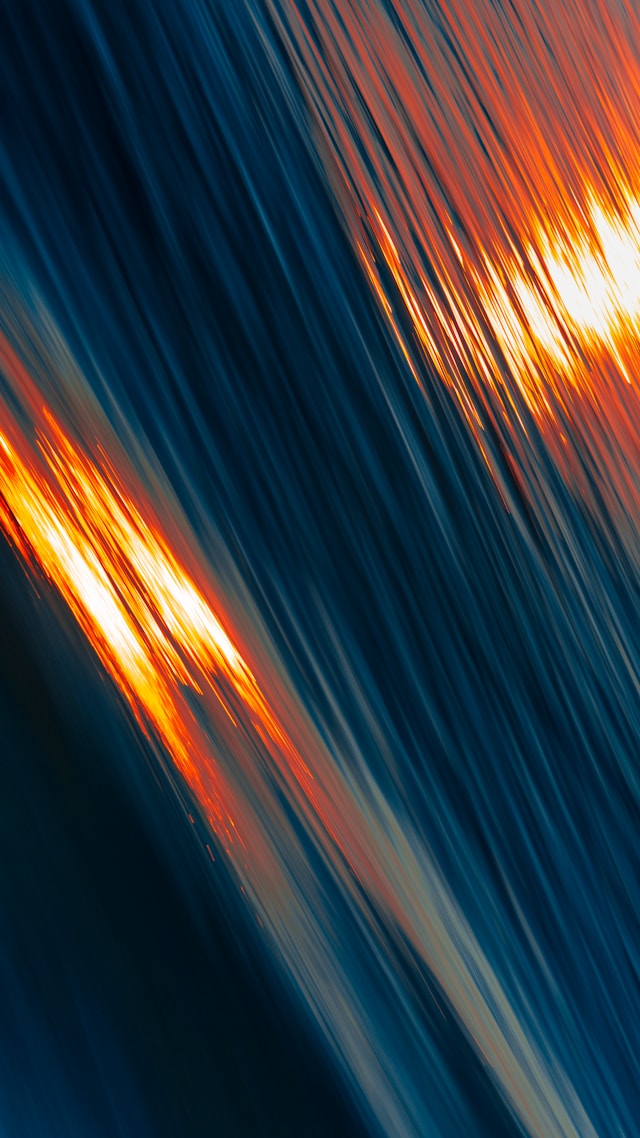
Understanding React Component Lifecycle and the Power of Common Components
React is a powerful JavaScript library for building dynamic user interfaces, and at the heart of its magic are components. Understanding the React component lifecycle is crucial for optimizing performance and managing state effectively. In this blog post, we'll delve into the React component lifecycle, explore key phases, and discuss the benefits of using common components across your applications.
React Component Lifecycle Overview
The React component lifecycle refers to the series of methods that are invoked in different stages of a component's existence. These stages include mounting, updating, and unmounting. By understanding and leveraging these lifecycle methods, developers can control what happens at each stage of a component's life, ensuring efficient rendering and smooth user experiences.
1. Mounting
The mounting phase is when a component is being inserted into the DOM. During this phase, the following lifecycle methods are typically called:
- constructor(): Used for initializing state and binding event handlers.
- render(): The only required method, responsible for rendering the component's JSX to the DOM.
- componentDidMount(): Invoked after the component is mounted, ideal for fetching data or interacting with external APIs.
2. Updating
The updating phase occurs when a component's state or props change, leading to a re-render. The key lifecycle methods in this phase include:
- shouldComponentUpdate(): Determines whether the component should re-render. Returning `false` can prevent unnecessary updates.
- componentDidUpdate(): Invoked after the component has been updated, useful for performing actions based on the previous state or props.
3. Unmounting
The unmounting phase is when a component is being removed from the DOM. The primary lifecycle method here is:
- componentWillUnmount(): Invoked before the component is unmounted and destroyed, perfect for cleaning up resources like timers, subscriptions, or listeners.
The Power of Common Components
In React, the concept of common components is fundamental to building reusable, maintainable, and consistent UI elements. Common components are those that are used across multiple parts of an application, such as buttons, forms, modals, and navigation bars. By creating a library of these components, developers can achieve a cohesive look and feel while reducing code duplication.
Advantages of Using Common Components
- Reusability: Common components can be used in various parts of your application, promoting code reuse and reducing redundancy.
- Consistency: By using common components, you ensure that your UI elements maintain a consistent appearance and behavior across your application.
- Maintainability: With a centralized set of components, updates and bug fixes can be applied in one place, simplifying maintenance and reducing the risk of errors.
- Customization: Common components can be designed with customization options, allowing them to be easily tailored to different contexts while maintaining core functionality.
Examples of Common Components
Here are some examples of common components that you might create in a React application:
- Button: A customizable button component with support for different styles, sizes, and states (e.g., primary, secondary, disabled).
- Input Field: An input component that handles various types of data (e.g., text, number, password) and includes validation and error handling.
- Modal: A reusable modal component that can display various types of content, such as forms, confirmations, or alerts.
- Card: A flexible card component that can be used to display content like images, text, and buttons in a consistent layout.
Conclusion
Mastering the React component lifecycle and leveraging common components are key practices for building efficient and maintainable applications. By understanding when and how to use lifecycle methods, you can optimize your components for performance and ensure smooth user experiences. Additionally, creating a library of common components allows you to build consistent, reusable UI elements that simplify development and maintenance. As you continue to develop with React, keep these concepts in mind to enhance your workflow and deliver high-quality applications.